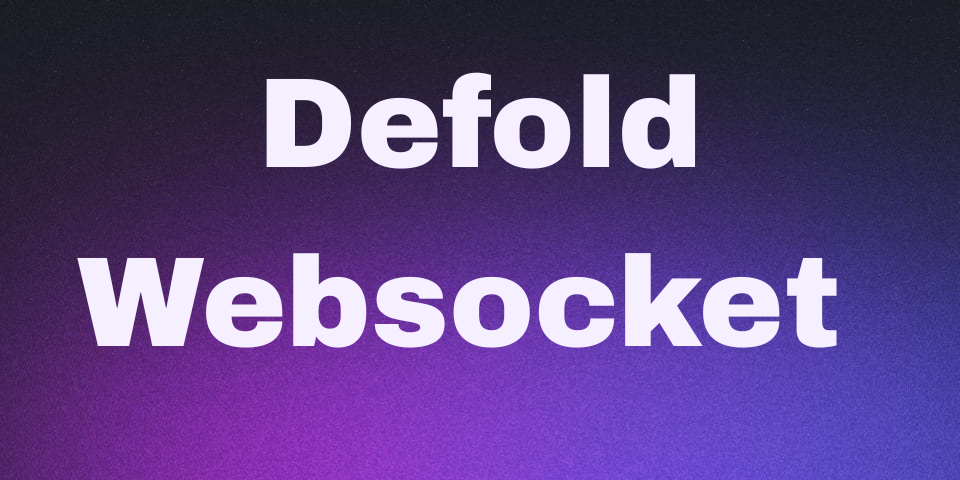
Defold: WebSocket Client Connection
Tested on defold 1.9.8 version
In this post i will show you how to add a simple client to defold made game/applicaiton.
In defold to be able to work with websocket connection ,there is a extension that you can use to create a websocket client connection.
Installation
To install the extension, you can use the Defold editor.
Open your game.project file in the Defold editor and go to the “Project” tab. Click the + button next to “Dependencies” and copy paste the following URL into the dialog:
For last version https://github.com/defold/extension-websocket/archive/master.zip
For special version
- Go to https://github.com/defold/extension-websocket/releases link and you will see the list of all the releases.
- Click on the release you want to use and copy the link to the zip file.
- Then paste the link into the dialog in the Defold editor.
For example : https://github.com/defold/extension-websocket/archive/refs/tags/4.0.0.zip
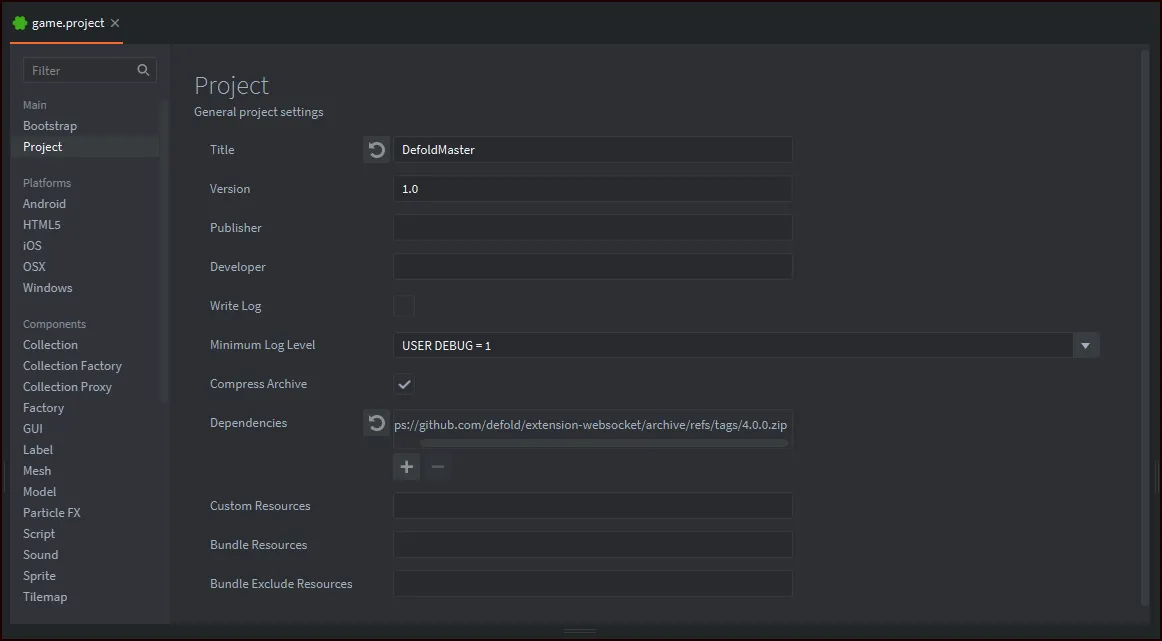
Then Go to Project -> Fetch Libraries and click on it. This will download the extension and add it to your project.
Configure
After you have installed the extension, you need to configure it in your game.project file. Open game.project file as text and add the following lines to the file:
[websocket]
debug = 1
socket_timeout = 10000000
Usage
You can use the websocket extension in your scripts. To use you dont need to import anything else just able to use websocket in your script.
First we need to connect to the server. Best place is for this init() function.
function init(self)
self.url = "ws://echo.websocket.org"
local params = {}
self.connection = websocket.connect(self.url, params, websocket_callback)
end
In the above code we are connecting to the echo websocket server. params can be use to pass timeout, headers and protocol.
websocket_callback is the function that will be called when the connection is established or when there is an error. So wee need to define that function before init function.
local function websocket_callback(self, conn, data)
if data.event == websocket.EVENT_DISCONNECTED then
print("Disconnected: " .. tostring(conn))
self.connection = nil
elseif data.event == websocket.EVENT_CONNECTED then
print("Connected: " .. tostring(conn))
elseif data.event == websocket.EVENT_ERROR then
print("Error: '" .. tostring(data.message) .. "'")
if data.handshake_response then
print("Handshake response status: '" .. tostring(data.handshake_response.status) .. "'")
for key, value in pairs(data.handshake_response.headers) do
print("Handshake response header: '" .. key .. ": " .. value .. "'")
end
print("Handshake response body: '" .. tostring(data.handshake_response.response) .. "'")
end
elseif data.event == websocket.EVENT_MESSAGE then
print("Receiving: '" .. tostring(data.message) .. "'")
end
end
After script remove for game cycle we need to remove the connection.So our server will know that we are not connected anymore.
function final(self)
if self.connection ~= nil then
websocket.disconnect(self.connection)
end
end
The information shared on DefoldMaster is based on my personal experience and learning process with the Defold game engine. While I strive to provide accurate and helpful content, mistakes may still occur. If you find any errors or inaccuracies in an article, please feel free to reach out to me from social accounts. Your feedback is greatly appreciated and helps improve the quality of the resources shared.